.NET SDK
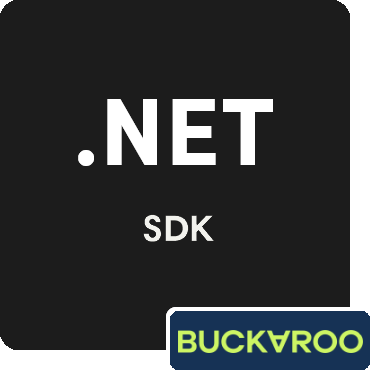
The Buckaroo .NET SDK is a comprehensive code library facilitating communication with the Buckaroo API. Standardizing requests with a fluent interface simplifies the process of initializing requests and managing common tasks such as authentication, signature calculation, logging, and generating JSON messages. This ensures that developers can focus on their core application logic without worrying about these details.
Download the Library
You can download the Buckaroo .NET SDK here:
SdkClient Class Setup
The SdkClient
class serves as the primary entry point for making requests using the Buckaroo SDK. Each function that interacts with the SDK requires an instance of this class. You can initialize this instance either in the constructor of your class or within each function as needed.
In our test suite, we initialize the SdkClient in the Setup() method:
internal SdkClient BuckarooClient { get; private set; }
[TestInitialize]
public void Setup()
{
this.BuckarooClient = new SdkClient();
}
Logging Options in SdkClient
The SdkClient class includes functionality for handling logging, addressing the challenge of understanding the internal workings of an external SDK. We've designed the Buckaroo SDK to be robust and hard to break, regardless of internal operations. Nevertheless, we have implemented logging functionality. The SDK offers two logging options: Standard and Extensive, and also allows for custom logger creation using the ILogger interface. The Logger can be specified either when creating the SdkClient or when making a request.
If no Logger is provided, as seen in the Setup() method above, the StandardLogger will be used by default.
// Example for a function that returns a custom implemented logger,
// which can be provided in the constructor like this:
// this.BuckarooClient = new SdkClient(TestSettings.Logger);
internal static Func<ILogger> Logger
{
get { return () => new CustomImplementationLogger(); }
}
Updated 4 months ago